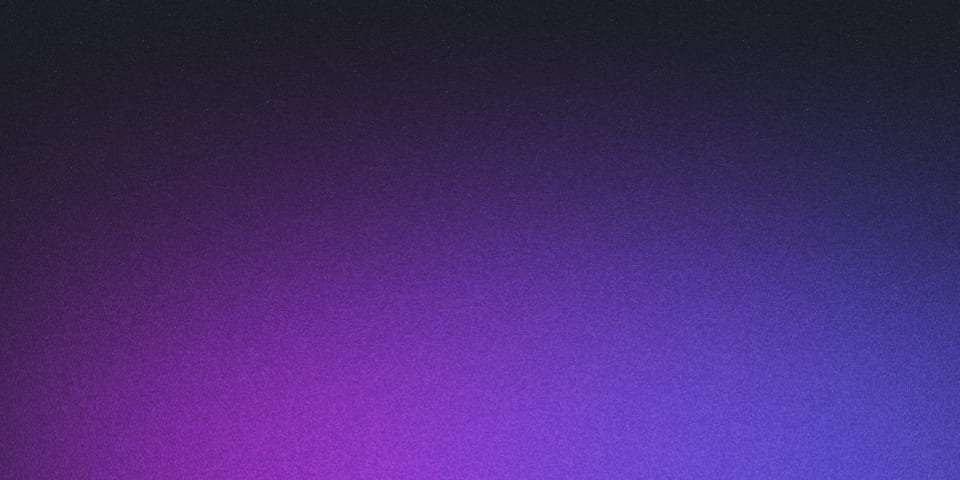
Import the Delicious dump into Pinboard
Import the Delicious dump into Pinboard
In a previous article, I explained how to dump all your bookmarks from Delicious using a web scraper. As I’m switching to Pinboard, I still needed a way to import the exported bookmarks.
Content structure
The exported bookmark file is a CSV file, but with a snippet of JSON for the bookmark tags:
"Lifehacker","http://lifehacker.com/","[{""tag"":""gtd""},{""tag"":""news""},{""tag"":""productivity""}]","tips and downloads for getting things done"
Import
The Pinboard API is fully documented online. To import the CSV file into Pinboard, I wrote a little Python script to process line by line.
import csv
import json
import urllib
bookmark_file = '<bookmark_file_from_delicious>'
pinboard_token = '<your pinboard token>'
def process_tags(csv_tags):
json_tags = json.loads('{ "tags": %s }' % csv_tags)
json_tags = json_tags['tags']
tags = []
for json_tag in json_tags:
tags.append(unicode(json_tag['tag']).encode('utf-8'))
return tags
with open(bookmark_file, 'rb') as csvfile:
bookmarks = csv.reader(csvfile, delimiter=',', quotechar='"')
next(bookmarks) # Skip header
for bookmark in bookmarks:
bookmark_post_data = {
'url': bookmark[1],
'description': bookmark[0]
}
if bookmark[3]!='null':
bookmark_post_data['extended'] = bookmark[3]
tags = process_tags(bookmark[2])
if len(bookmark[2]) > 0:
bookmark_post_data['tags'] = ",".join(tags)
bookmark_post_data['auth_token'] = pinboard_token
params = urllib.urlencode(bookmark_post_data)
print "Adding %s" % bookmark[1]
f = urllib.urlopen("https://api.pinboard.in/v1/posts/add?%s" % params)
Each line is converted from the CSV/JSON structure to a URL encoded set of arguments. These arguments are then used in an HTTP call to create the bookmark at Pinboard.
Run
Download a local copy of my script, update the variables at the beginning with the name of your dump file and put your Pinboard account token. You can find this token in the Pinboard Password settings.